15. Trees
CAUTION - CHAPTER UNDER CONSTRUCTION!
This chapter was last updated on May 7, 2025. Contents locked until 11:59 p.m. Pacific Standard Time on May 23, 2025.
A tree is a connected simple graph that contains no cycles. Trees are used to model decisions, to sort data, and to optimize networks.
Key terms and concepts covered in this chapter:
-
Trees
-
Properties
-
trees (binary, spanning)
-
expression trees
-
-
Traversal strategies
-
Spanning trees/forests
-
Spanning trees
-
tree traversals
15.1. Definitions, Examples, and Properties of Trees and Forests
Recall that a simple graph has no loops and no parallel edges, that is, no edge connects a vertex to itself, and no two edges can connect the same pair of vertices. In a simple graph, each edge is determined by its two vertices.
A tree is an undirected simple graph that is connected and has no cycles, which you may recall are paths that start and end at the same vertex. Some sources use the term acyclic to mean "has no cycles."
A forest is the union of several trees. A forest is a simple graph that has one or more connected components, where each connected component is a tree.
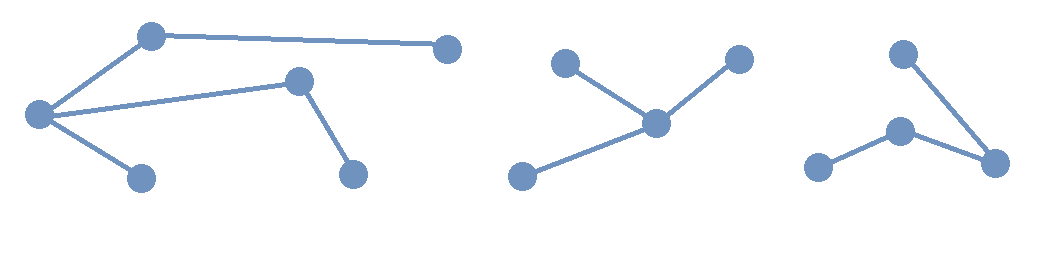
The image shows a forest composed of three trees.
Notice that if you choose any pair of vertices in one of the trees in the image, there is only one path that joins that pair of vertices. In fact, this property is True for any tree.
Here is another characterization of trees.
15.2. Additional Topics
Students in CSC 230 Spring 2025 can refer to the slide decks (from Spring 2024 and Fall 2024) that are posted in Canvas.
-
Spanning Trees and Spanning Forests
-
Kruskal’s Algorithm
-
-
Binary Trees
-
Tree Traversal Strategies
-
Expression Trees
-
-
Algorithms for Binary search trees
-
Algorithms for Depth- and breadth-first traversals
-